Docs
Everything You Need to Know
Widgets
Slide Out Menu
Slide out menus offer a convenient way to provide the user access to a number of otherwise unrelated screens. If you have more screens than can fit in a tab bar, but you don't want to use a navigation list in it, a slide out menu is a better option.
To create a slide out menu, you need to provide it with the screens you want to show when the menu items are tapped. First we create the slide out menu using the $.SlideOut
method. Then we populate the menu by passing it some options. These are an array of key/value pairs. The key is the id of the screens to use, and the value is the label that will be displayed in the slide out menu:
// Set up slide out menu: var appSlideOut = $.SlideOut(); // Populate slide out menu: appSlideOut.populate([ { music: 'Music' }, { pictures: 'Pictures' }, { documents: 'Documents'}, { recipes: 'Recipes' }, { favorites: 'Favorites' } ]);
In the above example, the ids of the screens will be 'music', 'pictures', 'docuemnts', 'recipes', and 'favorites'. And the slide menu labels will be 'Music', 'Pictures', etc. Tapping on the menu items will show the associated screen.
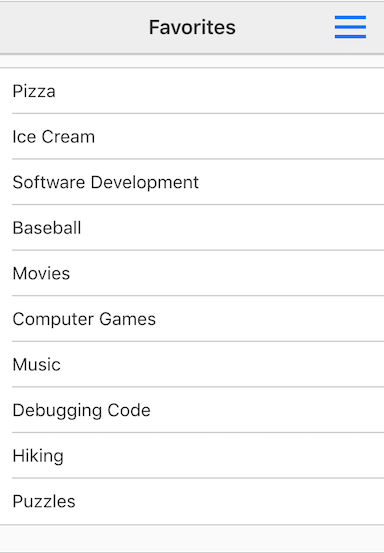
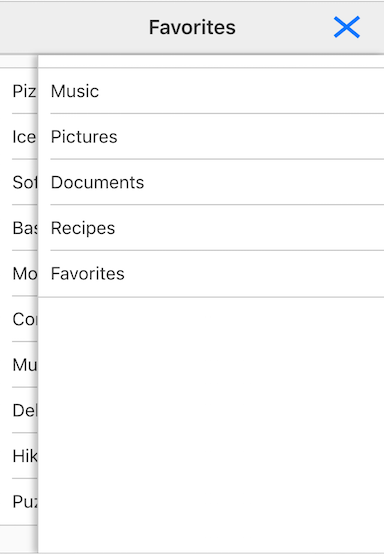
Routing
You can use routes with the slide out menu. You might do that when you want to have just one screen and render its contents depending on which menu item the user taps in the slide out. This is easy to do, even if the content is different because ChocolateChip-UI lets you switch out what template a view uses. Just as we set up routing in a navigation list by providing ids, we need to do the same here. ChocolateChip-UI automatically dispatches a route when the user taps a slide out menu, so you just need to provide each menu item with a unique id and set up a router to capture the dispatch. You may want to review ChocolateChip-UI's Router. Below we have set up a slide out to pass ids with the routes:
// Setup Slide Out: var appSlideOut = $.SlideOut(); // Notice names have `:` to // indicate parameter for routing. // Id will be used to render the view. appSlideOut.populate([ { "choice:music": 'Music' }, { "choice:pictures": 'Pictures' }, { "choice:documents": 'Documents'}, { "choice:recipes": 'Recipes' }, { "choice:favorites": 'Favorites' } ]);
Every menu item is showing the same screen, but passing a unique id with the route. We can capture that id with a router:
// Create the router: var MyRoutes = $.Router(); // Add a route: MyRoutes.addRoute([ { // The route: route: 'choice', // Callback to handle passed route parameter: callback: function(id) { // Method to render templates in switch statement: var renderChosenTemplate = function(element, template, item) { listView.setTemplate(template); listView.setElement(element); listView.render(item); }; // Handle passed id: switch (id) { case 'music': renderChosenTemplate('#myList', templates[0], music); break; case 'pictures': renderChosenTemplate('#gridOfImages', templates[1], imageCollection); break; case 'documents': renderChosenTemplate('#myList', templates[2], docs); break; case 'recipes': renderChosenTemplate('#myList', templates[3], recipes); break; case 'favorites': renderChosenTemplate('#myList', templates[4], favorites); break; } } } ]);
Slide Out Menu with Navigation List
You can also have a navigation list as one of the screens that a slide out menu reveals. It doesn't require anything special. Just put it there and provide its id to the slide out menu.
You can examine the following files in the examples folder of the source code to see working examples of how to set up slide out menus.
- slide-out-menu.html
- slide-out-menu-routes.html
- slide-out-menu-nav.html
Read the following instructions for getting the examples.
Slideout Example
See the Pen Slideout by Robert Biggs (@rbiggs) on CodePen.
Slide with Android Theme
See the Pen Slideout for Android by Robert Biggs (@rbiggs) on CodePen.