Docs
Everything You Need to Know
Widgets
Editable List
You may have a list that you want your users to be able to modify in some way, such as change the order or deleting some items. You can provide that functionality with ChocolateChip-UI's editable list. This allows you to make a list's items movable, or deletable, or both. You set this up when you initialize the list. Basically, an editable list is just a Component list with the ability to reorder and delete items.
Importing UIEditList Into Your Project
In order to make an editable list, you'll need to import the UIEditList class class into your project. You do that by by including the following at the top of your app.js
file in your project's dev
folder:
import {UIEditList} from './src/widgets/ui-editable'
After importing UIEditList, you are ready to ccreate an editable list.
You need to bind an editable list to state for it to work. That way, when the user is done modifying the list, its changes will to synched to the State object.
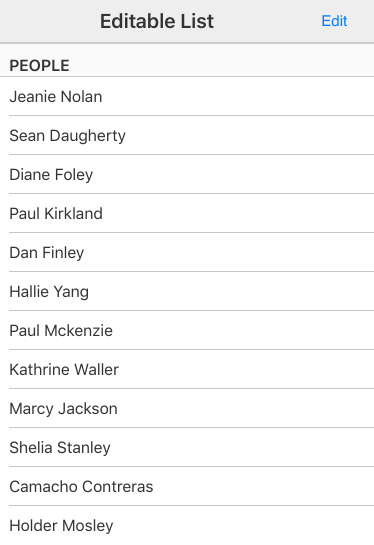
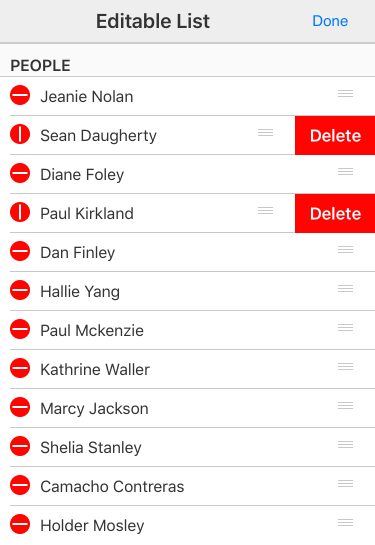
Moving a list item:
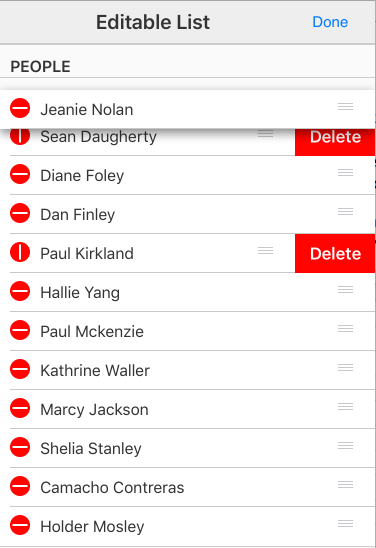
Below is an example of setting up an editable list:
const peopleState = new State(people) const editList = new UIEditList({ element: '#editList', state: peopleState, render: (person) => html` <li data-id='${ person.uuid }''> <h3>${ person.name.first } ${ person.name.last }</h3> </li>`, deletable: true, reorderable: true }) editList.render()
In this example we set the list items to be movable and deletable. We told the list instance what State object it should be bound to. We also provided a stateProp value, which in this case is a uuid. You need to use some value that is unique to each item in the State object. This allows ChocolateChip-UI to identify objects in the state to compare with the changes made by the user. We also need to indicate what view we used to render the list. We do that using the property data-id
in the editable list template, as shown above. data-id
value must be unique. This is used so that when items are deleted or moved, we can make the State object match. When you initialize a list to be editable, ChocolateChip-UI injects a number of elements into it that were not there when it was first rendered by the view, such as for moving or deleting list items. When you set a list to be reorderable, when the user taps the Edit
button, the list display the move handles (three lines stacked like a hamburger). When these are show, you can click with a mouse, or tap and hold with your finger. After a moment you will see the list item pop up from the list. Then you can drag it to the position you desire. You can click/tap anywhere on the list to drag, not just on the move handle.
You can get the current state of the editable list after changes were made by accessing its State object directly. In the above example we used a State object called peopleState
. After editing the list, we can get the latest state like so:
// Get lastest version of edit list data: const editListState = peopleState.val()
Because the editable list is bound to a State object, if you update the state, the editable list will update too.
Read the following instructions for getting the examples.
Editable List
See the Pen Editable List V5 by Robert Biggs (@rbiggs) on CodePen.
Editable List with Android Theme
See the Pen Editable List with Android Theme V5 by Robert Biggs (@rbiggs) on CodePen.