Docs
Everything You Need to Know
Widgets
Select List
When you need to provide the user the ability to pick one of several options, use ChocolateChip-UI's select list. Internally this is a radio button group, but visually it looks like a list of selectable items. Only one item can be selected at a time. If you need multi-select, use ChocolateChip-UI's Multi-Select List.
Importing UISelectList Into Your Project
In order to make a segmented button, you'll need to import the UISelectList class class into your project. You do that by by including the following at the top of your app.js
file in your project's dev
folder:
import {UISelectList} from './src/widgets/ui-select-list'
After importing UISelectList, you are ready to create a select button.
A select list needs an unordered list with the class list
as its base:
<ul class="list" id="selectList"></ul>
To turn that list into a select list, you need to define it with the following options: an element, a selected list item and a callback. If no selected item is provided, none of them will be selected at load time. You also need to define a template to use to render the list itself. The list expects pairs of value/label data to render.
// The select list expects // a set of value/label terms: const todosStore = new State([ {value: "eat", label: "Go Eat Something"}, {value: "nap", label: "Take a nap"}, {value: "work", label: "Get some work done"}, {value: "play", label: "Play a game"} ]) const selectList = new UISelectList({ element: '#my-list', selected: 2, name: 'my-list', state: todosStore, render: (todo) => html` <li data-select="${ todo.value}"> <h3>${ todo.label }</h3> </li>`, callback: () => renderSelection() })
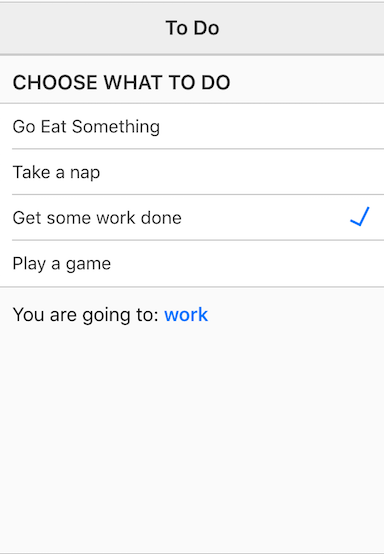
You can also provide a name option to use with the select list: name: 'myChoice'
. If you do not, defaults will be used. You would want to provide a name if you intend to get a JSON object from you form elements. Read about form validation to learn more. You can find out what the current selection of a list is by querying the instance of the list that you created. Please note that upto version 4.1.2 you would use getSelection()
, but since 4.1.3 the preferred way is to use value
:
const selectedValue = mySelectList.value
This will return currently selected input value of the list item.
If you want to get the index of the current selection, you can use:
// Get the selection index: const index = mySelectList.index
Please check out the file "selectlist.html" in the examples folder. Read the following instructions for getting the examples.
Select List Example
See the Pen Select List V5 by Robert Biggs (@rbiggs) on CodePen.
Selecti List with Android Theme
See the Pen Selecti List with Android Theme V5 by Robert Biggs (@rbiggs) on CodePen.