Docs
Everything You Need to Know
Widgets
Multi-Select List
Importing UIMultiSelectList Into Your Project
In order to make a multi-select list, you'll need to import the UIMultiSelectList class class into your project. You do that by by including the following at the top of your app.js
file in your project's dev
folder:
import {UIMultiSelectList} from './src/widgets/ui-multi-select-list'
After importing UIMultiSelectList, you are ready to create an multi-select list.
You can use ChocolateChip-UI's multi-select list to enable your users to select multiple items from a list. The setup is very similar to a select list. You create an instance and provide it with options:
const TODOS = [ {value: "eat", label: "Go Eat Something"}, {value: "nap", label: "Take a nap"}, {value: "work", label: "Get some work done"}, {value: "play", label: "Play a game"} ] const todoState = new State(TODOS) // Set up Select List: const myMulitSelectList = new UIMultiSelectList({ element: '#multiSelectList', selected: [1,2], name: 'personal_choice[]', state: todoState, render: (data) => ` <li> <h3>${data.label}</h3> </li>`, callback: () => renderSelection() }) myMulitSelectList.render() // Define callback for multi-select list: const renderSelection = function() { let temp = [] myMulitSelectList.selection.forEach((item) => temp.push(item.value)) temp.sort() temp = temp.join(', ') if (temp.length) { $('#response').text(temp) } else { $('#response').empty() } }
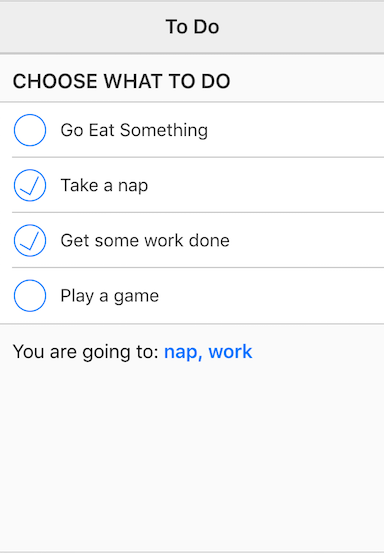
As you can see from the multi-select list options above, you can provide a series of pre-selected list items by using an array. Numbering is zero based. You can also provide a name option to use with the multi-select list: name: 'myChoice[]'
. You can see names being used in the Codepen examples below. If you do not, defaults will be used. You would want to provide a name if you intend to get a JSON object from your form elements. Read about form validation to learn more. To get the current value of a multi-select list, you use the selection
property on the list instance. This returns an array of objects like this:
const choices = myMulitSelectList.selection; // choices: /* [ {index: 2, value: "work"}, {index: 4, value: "vacation"} ] */
To evaluate the returned value, we can loop over it, as we are doing in the callback in the example above.
Please look at the file "multi-select-list.html" in the example folder. Read the following instructions for getting the examples.
Multi-Select List
See the Pen Multi-Select List V5 by Robert Biggs (@rbiggs) on CodePen.
Multi-Select List with Android Theme
See the Pen Multi-Select List with Android Theme V5 by Robert Biggs (@rbiggs) on CodePen.