Docs
Everything You Need to Know
Widgets
Sheets
Sheets are panels that slide down from the top of the app, or up from the bottom. They completely cover the screen but are semi-transparent so the user can see what is behind. On Android and iOS the content behind the sheet gets blurred.
Importing UISheet Into Your Project
In order to make a segmented button, you'll need to import the UISheet class class into your project. You do that by by including the following at the top of your app.js
file in your project's dev
folder:
import {UISheet} from './src/widgets/ui-sheet'
After importing UISheet, you are ready to create a sheet.
We can initialize a sheet with three options: an id, whether to show the default handle or not, and whether it slides down or up. By default, sheets have a handle along the top and they slide up from the bottom:
const sheet = new UISheet({ id: 'mySheet', handle: true, slideFrom: 'top' })
You can tell the sheet where to slide from: top or bottom using the slideFrom
property. In the above example we gave it "top", but you might prefer "bottom". Up to you where you what the sheet to slide in from.
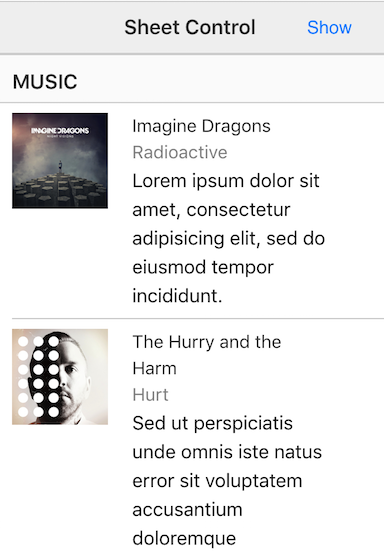
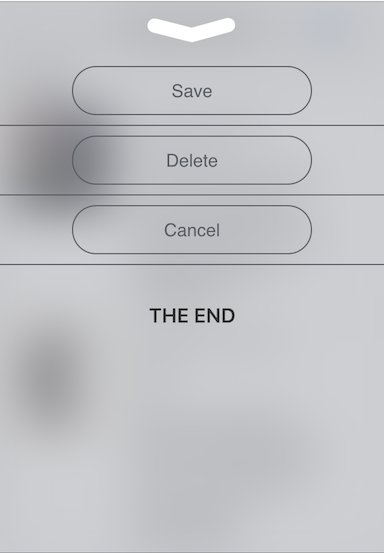
The above code creates the sheet. But it is currently empty. We need to populate it with things the user can interact with. It's your choice what you want to put in a sheet. In the following code we are going to populate the shet and attach an event to handle user interaction:
sheet.populate(`<ul class='list'> <li><button class='action'>Save</button></li> <li><button class='action'>Delete</button></li> <li><button class='action'>Cancel</button></li> </ul> <h2 style="text-align: center; margin: 20px;">The End</h2>`) // Hide sheet when tapping button: $('#mySheet').on('tap', 'button', function() { sheet.hide(); })
If we were doing this properly, we would delegate events on the sheet to handle the buttons. You get the idea.
Finally, we need an event to show the sheet:
// Initialize button to show sheet: $('#showSheet').on('tap', function() { $.ShowSheet('#mySheet'); });
Check out the file "sheets.html" in the examples folder. Read the following instructions for getting the examples.
Sheets Example
See the Pen Sheets V5 by Robert Biggs (@rbiggs) on CodePen.
Sheets with Android Theme
See the Pen Sheets Android Theme V5 by Robert Biggs (@rbiggs) on CodePen.