Docs
Everything You Need to Know
Widgets
Switches
ChocolateChip-UI has switches. These are controls for turning things on or off, or for other either/or situations in an interface. Internally they are simply checkboxes with a checked or unchecked state.
Importing UISwitch Into Your Project
In order to make a segmented button, you'll need to import the UISwitch class class into your project. You do that by by including the following at the top of your app.js
file in your project's dev
folder:
import {UISwitch} from './src/widgets/ui-switch'
After importing UISwitch, you are ready to create a switch control.
To create a switch, you first need to have a ui-switch tag in the document:
<ui-switch id='sleep-switch'></ui-switch>
To make the switch look and act properly, you need to initialize it:
const sleepSwitch = new UISwitch({ element: '#sleep-switch', name: 'sleep', value: 'get some sleep', checked: false, on: () => { $('#switchCallbacks').text('On callback: Just turned switch one on!') $('#response').text(sleepSwitch.val().value) console.log(sleepSwitch.val()) }, off: () => { $('#switchCallbacks').text('Off callback: Turned switch one off!') $('#response').empty() } })
Notice: You can use both on
and off
callbacks for a switch. Which you use depends on what action you want to catpure.
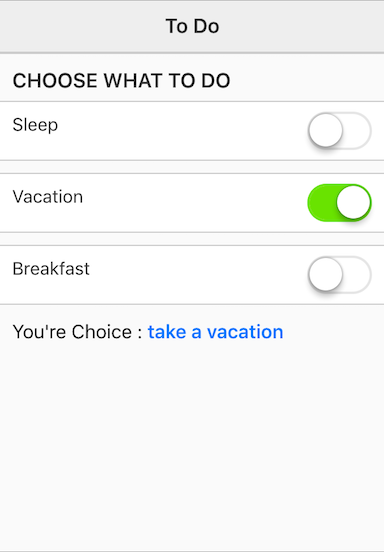
In the above example notice that we provided the id of the switch to initialize. The name value will be used as the name of the switch's checkbox. The value will be the value of the checkbox. The checked value will set the initalial state of the switch and checkbox. And finally, we provide two callbacks: onCallback and offCallback. You don't have to provide both. Just use the one you are interested in, or you might want to use both. It's your choice. We can get the value of any switch by accessing the value
property on the switch instance.
const value = mySwitch.value
You can verify whether a switch is checked/on or unchecked/off by examining the switch instance's checked
property:
const checked = sleepSwitch.checked // Gets the checked state: true or false
Check out the demo of switches in the example folder. Read the following instructions for getting the examples.
iOS Switches Example
See the Pen iOS Switches V5 by Robert Biggs (@rbiggs) on CodePen.
Android Switches Example
See the Pen Android Switches V5 by Robert Biggs (@rbiggs) on CodePen.